Separate ChatGPT inputs to chunks and save it to clipboard
chatGPT, prompt engineering
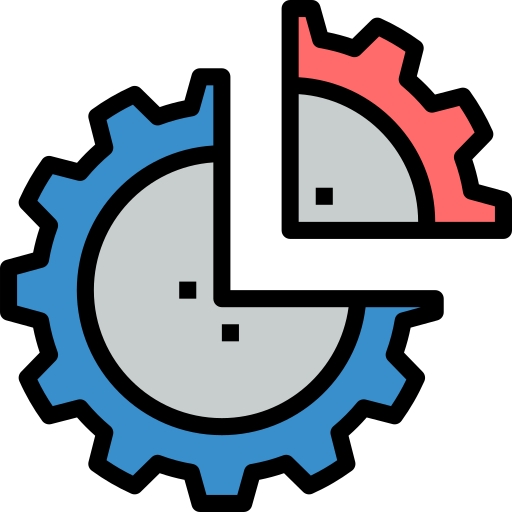
Prerequirements
First of all , you need a clipboard manager (like
alfred5
) to use it effectively
Example
Run command
bun src/index.ts
Then copy every content with the given order to ChatGPT
bun src/index.ts
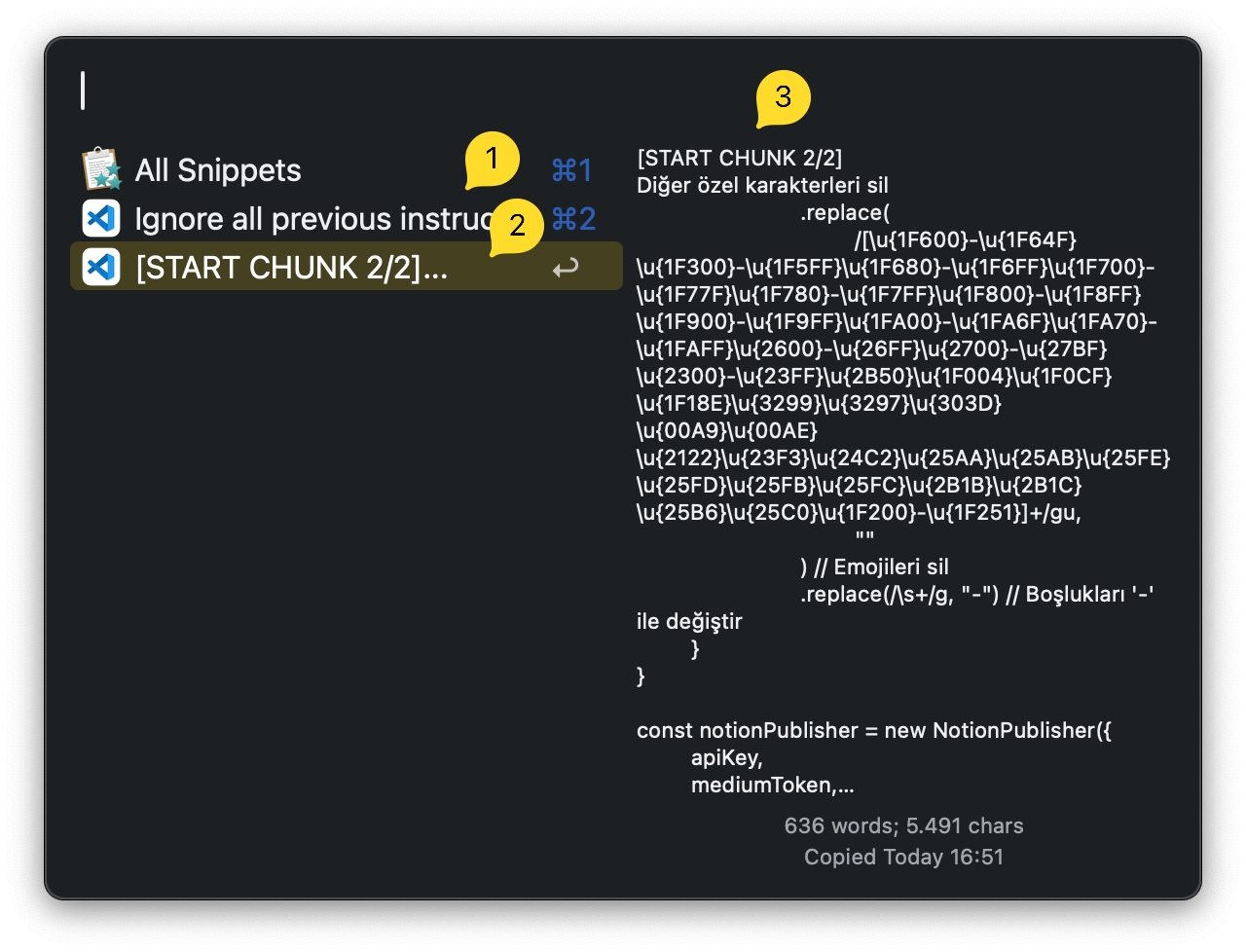
Index.ts
/**
* Processes given content to prepare for ChatGPT.
* @param content - The content to process.
* @returns Processed content chunks.
*/
export function processContentForChatGPT(content: string): string[] {
const chunks = separateTextForChatGPT(content)
const processedChunks = processChunksForChatGPT(chunks)
return processedChunks
}
/**
* Processes given file to prepare for ChatGPT.
* @param filePath - The path of the file.
* @returns Processed file content chunks.
*/
export async function processFileForChatGPT(filePath: string): Promise<string[]> {
const chunks = await separateFilesForChatGPT(filePath)
const processedChunks = processChunksForChatGPT(chunks)
return processedChunks
}
/**
* Separates given text into chunks.
* @param text - The text to separate.
* @param chunkSize - The size of each chunk. Default is 8000.
* @returns Text chunks.
*/
export function separateTextForChatGPT(text: string, chunkSize = 8000): string[] {
const numChunks = Math.ceil(text.length / chunkSize)
return Array.from({ length: numChunks }, (_, i) => {
const start = i * chunkSize
return text.slice(start, start + chunkSize)
})
}
/**
* Separates content of given file into chunks.
* @param filePath - The path of the file.
* @param chunkSize - The size of each chunk. Default is 8000.
* @returns File content chunks.
*/
export async function separateFilesForChatGPT(
filePath: string,
chunkSize = 8000
): Promise<string[]> {
return Bun.file(filePath)
.text()
.then(fileContent => separateTextForChatGPT(fileContent, chunkSize))
}
/**
* Adds additional instructions to given chunks.
* @param chunks - The chunks to process.
* @returns Processed chunks with added instructions.
*/
export function processChunksForChatGPT(chunks: string[]): string[] {
if (chunks.length > 0) {
chunks[0] =
"Ignore all previous instructions before this one. " +
"Act like a document/text loader until you load and remember content of the next text/s or document/s.\n" +
"There might be multiple files, each file is marked by name in the format ### DOCUMENT NAME.\n" +
"I will send you them by chunks. Each chunk start will be noted as [START CHUNK x/TOTAL], " +
"and end of this chunk will be noted as [END CHUNK x/TOTAL], " +
" where x is number of current chunk and TOTAL is number of all chunks I will send you.\n" +
"I will send you multiple messages with chunks, for each message just reply OK: [CHUNK x/TOTAL], don't reply anything else, don't explain the text!\n" +
`Let's begin:\n[START CHUNK 1/${chunks.length}]\n${chunks[0]}`
}
return chunks.map((chunk, index) => {
const chunkNum = index + 1
if (chunkNum === 1) {
return (
`${chunk}\n[END CHUNK ${chunkNum}/${chunks.length}]\n` +
"Reply with OK: [CHUNK x/TOTAL], DON'T reply anything else, DON'T explain the text, DON'T do anything else until i tell you otherwise!"
)
} else {
return (
`[START CHUNK ${chunkNum}/${chunks.length}]\n${chunk}\n[END CHUNK ${chunkNum}/${chunks.length}]\n` +
"Reply with OK: [CHUNK x/TOTAL], DON'T reply anything else, DON'T explain the text, DON'T do anything else until i tell you otherwise!"
)
}
})
}
import clipboard from "clipboardy"
/**
* Copies multiple strings to clipboard one by one.
* @param text - The text chunks to copy to clipboard.
*/
export async function copyToClipboard(...text: string[]) {
for (const chunk of text) {
await clipboard.write(chunk)
await Bun.sleep(100)
}
}
/**
* Reads content from clipboard.
* @returns Content currently in clipboard.
*/
export async function readClipboard() {
return await clipboard.read()
}
const stringifiedClipboard = await readClipboard()
const processedChunks = processContentForChatGPT(stringifiedClipboard)
await copyToClipboard(...processedChunks.reverse())
Last updated
Was this helpful?